Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 01BFS
- PS
- 엘라스틱서치
- 네트워크플로우
- 완전탐색
- 알고리즘
- 백준
- 구현
- 재귀함수
- DFS
- dp
- COSPRO
- 다익스트라
- 자바PS
- java
- BFS
- deque
- COSPROJAVA1급
- 시뮬레이션
- 이젠 골드구현도 어렵네..
- 게더타운시작
- YBMCOS
- 우선순위큐
- 취득후기
- spring
- QUICKSTARTGUIDE
- 세그먼트트리
- 백준코딩테스트
- GatherTown
- 다이나믹프로그래밍
Archives
- Today
- Total
공부공간
BOJ - 4386 ) 별자리 만들기 본문
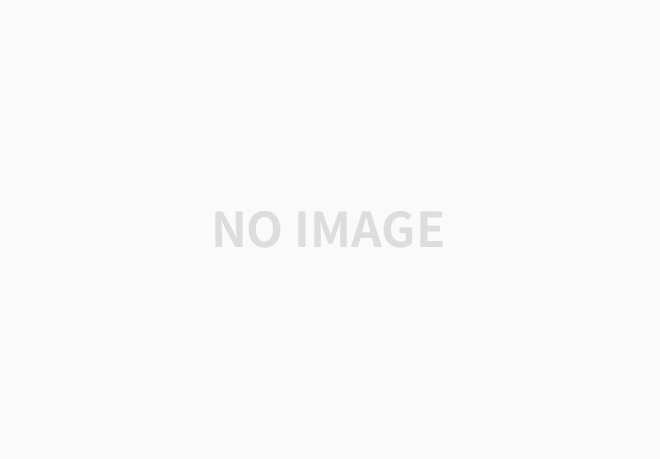
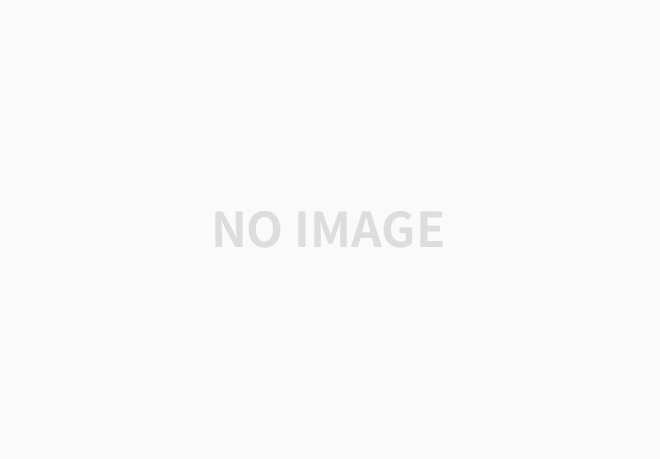
4386번: 별자리 만들기
도현이는 우주의 신이다. 이제 도현이는 아무렇게나 널브러져 있는 n개의 별들을 이어서 별자리를 하나 만들 것이다. 별자리의 조건은 다음과 같다. 별자리를 이루는 선은 서로 다른 두 별을 일
www.acmicpc.net
별자리를 클래스로 묶고 별자리 간 거리를 계산해서 우선순위 큐에 넣는다.
거리가 짧은 별자리들의 쌍이 항상 나오므로, 크루스칼 알고리즘으로 MST를 완성한다.
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.util.Comparator;
import java.util.PriorityQueue;
import java.util.StringTokenizer;
public class 별자리만들기 {
public static class star{
double x,y;
int num;
public star(double x, double y , int num) {
super();
this.x = x;
this.y = y;
this.num =num;
}
}
public static class dist{
int s ,e ;
double distance;
public dist(int s, int e, double distance) {
super();
this.s = s;
this.e = e;
this.distance = distance;
}
}
public static int find(int x) {
if(x==parent[x]) return x;
else return parent[x] = find(parent[x]);
}
public static void union (int pa , int pb) {
if(pa > pb) {
int t = pb;
pb = pa;
pa = t;
}
parent[pa] = pb;
return;
}
public static int parent[];
public static void main(String[] args) throws Exception {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine());
int n = Integer.parseInt(st.nextToken());
star list[] = new star[n];
parent = new int[n];
for(int i=0;i<n;i++) parent[i] =i;
for(int i =0 ; i < n ; i++) {
st = new StringTokenizer(br.readLine());
double xx = Double.parseDouble(st.nextToken());
double yy = Double.parseDouble(st.nextToken());
list[i] = new star(xx, yy, i);
}
PriorityQueue<dist> pq = new PriorityQueue<>(new Comparator<dist>() {
@Override
public int compare(dist o1, dist o2) {
if(o1.distance > o2.distance) return 1;
else if(o1.distance == o2.distance) return 0;
else {
return -1;
}
}
});
for(int i =0; i< n ; i ++) {
for(int j=i+1 ; j < n ; j ++) {
pq.add(new dist(i, j, Math.sqrt((list[i].x - list[j].x)*(list[i].x - list[j].x) + (list[i].y - list[j].y)*(list[i].y - list[j].y))));
}
}
double answer =0;
int cnt =1;
while(cnt < n) {
dist now = pq.poll();
int pa = find(now.s);
int pb = find(now.e);
if(pa !=pb) {
cnt++;
union(pa, pb);
answer += now.distance;
}
}
System.out.println(answer);
}
}
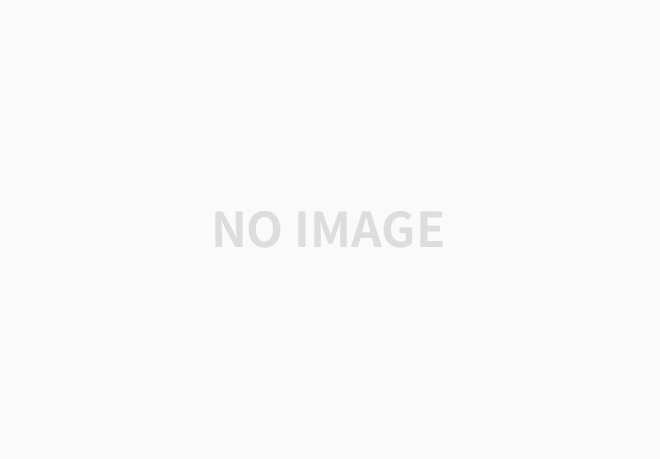